The C language programming. Patterns
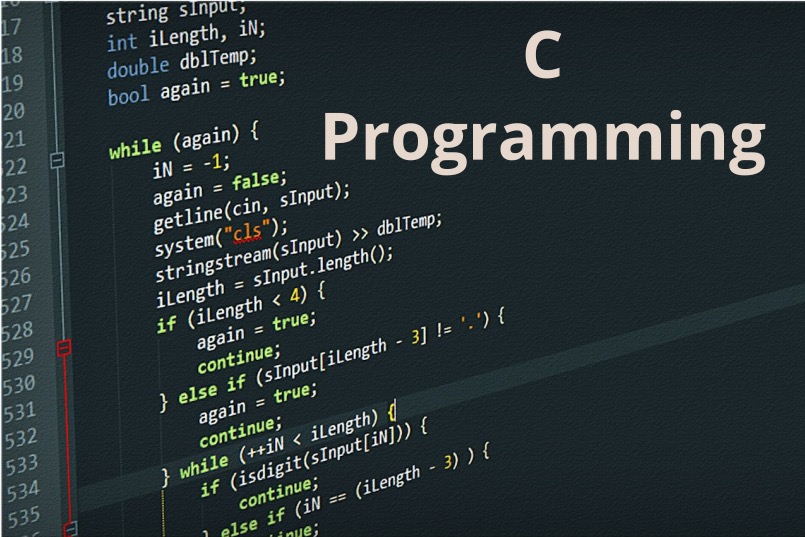
Patterns in C programming
Here will learn to print half pyramids, inverted pyramids, full pyramids, inverted full pyramids, Pascal's triangle, and Floyd's triangle in C Programming.
To understand this example, you should know about the following C programming topics:
1. C if...else Statement
2. C for Loop
3. C while and do...while Loop
4. C break and continue
Full square of *
Enter the number of rows:
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
Code
#include <stdio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i) {
for (j = 1; j <= rows; ++j) {
printf("* ");
}
printf("*");
}
Half Pyramid of *
Enter the number of rows:
*
* *
* * *
* * * *
* * * * *
Code
#include <stdio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i) {
for (j = 1; j <= i; ++j) {
printf("* ");
}
printf("\n");
}
return 0;
}
Half reverse Pyramid of *
Enter the number of rows:
* * * * *
* * * *
* * *
* *
*
Code
#include <stdio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = rows; i >= 1; --i) {
for (j = i; j >= 1; --j) {
printf("* ");
}
printf("\n");
}
return 0;
}
Full Pyramid of *
*
* * *
* * * * *
* * * * * * *
Code
#include <stdio.h>
int main() {
int i, space, rows, k = 0;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i, k = 0) {
for (space = 1; space <= rows - i; ++space) {
printf(" ");
}
while (k != 2 * i - 1) {
printf("* ");
++k;
}
printf("\n");
}
return 0;
}
Inverted full pyramid of *
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
Code
#include <stdio.h>
int main() {
int rows, i, j, space;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = rows; i >= 1; --i) {
for (space = 0; space < rows - i; ++space)
printf(" ");
for (j = i; j <= 2 * i - 1; ++j)
printf("* ");
for (j = 0; j < i - 1; ++j)
printf("* ");
printf("\n");
}
return 0;
}
Hollow rectangle
Enter the number of rows: 4
Enter the number of columns: 6
Output -
* * * * * *
* *
* *
* * * * * *
Code
#include <stdio.h>
void hollow_rectangle(int n, int m)
{
int i, j;
for (i = 1; i <= n; i++)
{
for (j = 1; j <= m; j++)
{
if (i==1 || i==n || j==1 || j==m)
printf(“*”);
else
printf(” “);
}
printf(“n”);
}
}
int main()
{
int rows, columns;
printf(“nEnter the number of rows : “);
scanf(“%d”, &rows);
printf(“nEnter the number of columns : “);
scanf(“%d”, &columns);
printf(“n”);
hollow_rectangle(rows, columns);
return 0;
}
Full kite
Enter the number of rows: 5
*
***
*****
*******
*********
*******
*****
***
*
Code
#include <stdio.h>
int main()
{
int n, c, k, space = 1;
printf(“Enter the number of rows\n”);
scanf(“%d”, &n);
space = n – 1;
for (k = 1; k <= n; k++)
{
for (c = 1; c <= space; c++)
printf(” “);
space–;
for (c = 1; c <= 2*k-1; c++)
printf(“*”);
printf(“\n”);
}
space = 1;
for (k = 1; k <= n – 1; k++)
{
for (c = 1; c <= space; c++)
printf(” “);
space++;
for (c = 1 ; c <= 2*(n-k)-1; c++)
printf(“*”);
printf(“\n”);
}
return 0;
}
Side triangle
Enter the number of rows: 5
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
Code
#include
int main()
{
int i, j, space, k = 0, n;
printf(“\nEnter the number of rows : “);
scanf(“%d”,&n);
for(int i=1;i<=n;i++)
{
for(int j=1;j<=n-i;j++)
{
printf(” “);
}
for(int j=1;j<=i;j++)
{
printf(“*”);
}
printf(“\n”);
}
for(int i=n-1;i>0;i–)
{
for(int j=1;j<=n-i;j++)
{
printf(” “);
}
for(int j=1;j<=i;j++)
{
printf(“*”);
}
printf(“\n”);
}
}
Number in rectangle
Enter the number of rows: 5
Enter the number of columns: 5
11111
22222
33333
44444
55555
Code
#include <stdio.h>
int main()
{
int rows, cols, i, j;
printf("Enter number of rows: ");
scanf("%d", &rows);
printf("Enter number of columns: ");
scanf("%d", &cols);
for(i=1; i<=rows; i++)
{
for(j=1; j<=cols; j++)
{
// Print the current row number
printf("%d", i);
}
printf("\n");
}
return 0;
}
Number in Rectangle
Enter the number of rows: 5
Enter the number of columns: 5
12345
12345
12345
12345
12345
Code
#include <stdio.h>
int main()
{
int rows, cols, i, j;
printf("Enter number of rows: ");
scanf("%d", &rows);
printf("Enter number of columns: ");
scanf("%d", &cols);
for(i=1; i<=rows; i++)
{
for(j=1; j<=cols; j++)
{
printf("%d", j);
}
printf("\n");
}
return 0;
}
Number switching rectangle
Enter N: 5
12345
21234
32123
43212
54321
Code
#include <stdio.h>
int main()
{
int N, i, j;
printf("Enter N: ");
scanf("%d", &N);
for(i=1; i<=N; i++)
{
for(j=i; j>1; j--)
{
printf("%d", j);
}
for(j=1; j<= (N-i +1); j++)
{
printf("%d", j);
}
printf("\n");
}
return 0;
}
Revers order number in square
Enter the number of rows: 5
Enter the number of columns: 5
12345
23451
34521
45321
54321
Code
#include <stdio.h>
int main()
{
int rows, cols, i, j;
printf("Enter number of rows: ");
scanf("%d", &rows);
printf("Enter number of columns: ");
scanf("%d", &cols);
for(i=1; i<=rows; i++)
{
for(j=i; j<=cols; j++)
{
printf("%d", j);
}
for(j=i-1; j>=1; j--)
{
printf("%d", j);
}
printf("\n");
}
return 0;
}
Half Pyramid of Numbers
Enter the number of rows:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Code
#include <stdio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i) {
for (j = 1; j <= i; ++j) {
printf("%d ", j);
}
printf("\n");
}
return 0;
}
Inverted half pyramid of numbers
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Code
#include <stdio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = rows; i >= 1; --i) {
for (j = 1; j <= i; ++j) {
printf("%d ", j);
}
printf("\n");
}
return 0;
}
Half Pyramid of Alphabets
A
B B
C C C
D D D D
E E E E E
Code
#include <stdio.h>
int main() {
int i, j;
char input, alphabet = 'A';
printf("Enter an uppercase character you want to print in the last row: ");
scanf("%c", &input);
for (i = 1; i <= (input - 'A' + 1); ++i) {
for (j = 1; j <= i; ++j) {
printf("%c ", alphabet);
}
++alphabet;
printf("\n");
}
return 0;
}
Full Pyramid of Numbers
1
2 3 2
3 4 5 4 3
4 5 6 7 6 5 4
5 6 7 8 9 8 7 6 5
Code
#include <stdio.h>
int main() {
int i, space, rows, k = 0, count = 0, count1 = 0;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; ++i) {
for (space = 1; space <= rows - i; ++space) {
printf(" ");
++count;
}
while (k != 2 * i - 1) {
if (count <= rows - 1) {
printf("%d ", i + k);
++count;
} else {
++count1;
printf("%d ", (i + k - 2 * count1));
}
++k;
}
count1 = count = k = 0;
printf("\n");
}
return 0;
}
Pascal's Triangle
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
Code
#include <stdio.h>
int main() {
int rows, coef = 1, space, i, j;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 0; i < rows; i++) {
for (space = 1; space <= rows - i; space++)
printf(" ");
for (j = 0; j <= i; j++) {
if (j == 0 || i == 0)
coef = 1;
else
coef = coef * (i - j + 1) / j;
printf("%4d", coef);
}
printf("\n");
}
return 0;
}
Floyd's Triangle.
1
2 3
4 5 6
7 8 9 10
Code
#include <stdio.h>
int main() {
int rows, i, j, number = 1;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; i++) {
for (j = 1; j <= i; ++j) {
printf("%d ", number);
++number;
}
printf("\n");
}
return 0;
}
Hope this found you informative and help full.
Great work
ReplyDelete